You are using an outdated browser. Upgrade your browser today or install Google Chrome Frame to better experience this site.
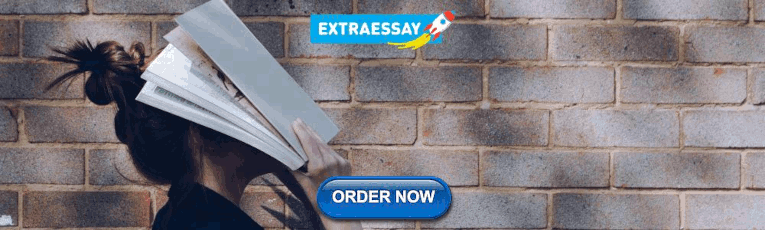
Learn X in Y minutes
Where x=prolog.
Get the code: learnprolog.pl
Prolog is a logic programming language first specified in 1972, and refined into multiple modern implementations.
Ready For More?
Got a suggestion? A correction, perhaps? Open an Issue on the GitHub Repo, or make a pull request yourself!
Originally contributed by hyphz, and updated by 4 contributors .
Facts A fact is a predicate expression that makes a declarative statement about the problem domain. Whenever a variable occurs in a Prolog expression, it is assumed to be universally quantified . Note that all Prolog sentences must end with a period. likes(john, susie). /* John likes Susie */ likes(X, susie). /* Everyone likes Susie */ likes(john, Y). /* John likes everybody */ likes(john, Y), likes(Y, john). /* John likes everybody and everybody likes John */ likes(john, susie); likes(john,mary). /* John likes Susie or John likes Mary */ not(likes(john,pizza)). /* John does not like pizza */ likes(john,susie) :- likes(john,mary)./* John likes Susie if John likes Mary. Rules A rule is a predicate expression that uses logical implication (:-) to describe a relationship among facts. Thus a Prolog rule takes the form left_hand_side :- right_hand_side . This sentence is interpreted as: left_hand_side if right_hand_side . The left_hand_side is restricted to a single, positive, literal , which means it must consist of a positive atomic expression. It cannot be negated and it cannot contain logical connectives. This notation is known as a Horn clause . In Horn clause logic, the left hand side of the clause is the conclusion, and must be a single positive literal. The right hand side contains the premises. The Horn clause calculus is equivalent to the first-order predicate calculus. Examples of valid rules: friends(X,Y) :- likes(X,Y),likes(Y,X). /* X and Y are friends if they like each other */ hates(X,Y) :- not(likes(X,Y)). /* X hates Y if X does not like Y. */ enemies(X,Y) :- not(likes(X,Y)),not(likes(Y,X)). /* X and Y are enemies if they don't like each other */ Examples of invalid rules: left_of(X,Y) :- right_of(Y,X) /* Missing a period */ likes(X,Y),likes(Y,X) :- friends(X,Y). /* LHS is not a single literal */ not(likes(X,Y)) :- hates(X,Y). /* LHS cannot be negated */ Queries The Prolog interpreter responds to queries about the facts and rules represented in its database. The database is assumed to represent what is true about a particular problem domain. In making a query you are asking Prolog whether it can prove that your query is true. If so, it answers "yes" and displays any variable bindings that it made in coming up with the answer. If it fails to prove the query true, it answers "No". Whenever you run the Prolog interpreter, it will prompt you with ?- . For example, suppose our database consists of the following facts about a fictitious family. father_of(joe,paul). father_of(joe,mary). mother_of(jane,paul). mother_of(jane,mary). male(paul). male(joe). female(mary). female(jane). We get the following results when we make queries about this database. (I've added comments, enclosed in /*..*/, to interpret each query.) Script started on Wed Oct 01 14:29:32 2003 sh-2.05b$ gprolog GNU Prolog 1.2.16 By Daniel Diaz Copyright (C) 1999-2002 Daniel Diaz | ?- ['family.pl']. compiling /home/ram/public_html/cpsc352/prolog/family.pl for byte code... /home/ram/public_html/cpsc352/prolog/family.pl compiled, 9 lines read - 999 bytes written, 94 ms (10 ms) yes | ?- listing. mother_of(jane, paul). mother_of(jane, mary). male(paul). male(joe). female(mary). female(jane). father_of(joe, paul). father_of(joe, mary). (10 ms) yes | ?- father_of(joe,paul). true ? yes | ?- father_of(paul,mary). no | ?- father_of(X,mary). X = joe yes | ?- Prolog interruption (h for help) ? h a abort b break c continue e exit d debug t trace h/? help Prolog interruption (h for help) ? e sh-2.05b$ exit script done on Wed Oct 01 14:30:50 2003 Closed World Assumption. The Prolog interpreter assumes that the database is a closed world -- that is, if it cannot prove something is true, it assumes that it is false. This is also known as negation as failure -- that is, something is false if PROLOG cannot prove it true given the facts and rules in its database. In this case, in may well be (in the real world), that Paul is the father of Mary, but since this cannot be proved given the current family database, Prolog concludes that it is false. So PROLOG assumes that its database contains complete knowledge of the domain it is being asked about. Prolog's Proof Procedure
8.21 Global variables
- Introduction
- g_assign/2 , g_assignb/2 , g_link/2
- g_array_size/2
- g_inc/3 , g_inc/2 , g_inco/2 , g_inc/1 , g_dec/3 , g_dec/2 , g_deco/2 , g_dec/1
- g_set_bit/2 , g_reset_bit/2 , g_test_set_bit/2 , g_test_reset_bit/2
8.21.1 Introduction
GNU Prolog provides a simple and powerful way to assign and read global variables. A global variable is associated with each atom, its initial value is the integer 0. A global variable can store 3 kinds of objects:
- a copy of a term (the assignment can be made backtrackable or not).
- a link to a term (the assignment is always backtrackable).
- an array of objects (recursively).
The space necessary for copies and arrays is dynamically allocated and recovered as soon as possible. For instance, when an atom is associated with a global variable whose current value is an array, the space for this array is recovered (unless the assignment is to be undone when backtracking occurs).
When a link to a term is associated with a global variable, the reference to this term is stored and thus the original term is returned when the content of the variable is read.
Global variable naming convention : a global variable is referenced by an atom.
If the variable contains an array, an index (ranging from 0) can be provided using a compound term whose principal functor is the corresponding atom and the argument is the index. In case of a multi-dimensional array, each index is given as the arguments of the compound term.
If the variable contains a term (link or copy), it is possible to only reference a sub-term by giving its argument number (also called argument selector). Such a sub-term is specified using a compound term whose principal functor is -/2 and whose first argument is a global variable name and the second argument is the argument number (from 1). This can be applied recursively to specify a sub-term of any depth. In case of a list, a argument number I represents the Ith element of the list. In the rest of this section we use the operator notation since - is a predefined infix operator (section 8.14.10 ).
In the following, GVarName represents a reference to a global variable and its syntax is as follows:
When a GVarName is used as an index or an argument number (i.e. indirection), the value of this variable must be an integer.
Here are some examples of the naming convention:
Here are the errors associated with global variable names and common to all predicates.
Arrays : the predicates g_assign/2 , g_assignb/2 and g_link/2 (section 8.21.2 ) can be used to create an array. They recognize some terms as values. For instance, a compound term with principal functor g_array is used to define an array of fixed size. There are 3 forms for the term g_array :
- g_array(Size) : if Size is an integer > 0 then defines an array of Size elements which are all initialized with the integer 0 .
- g_array(Size, Initial) : as above but the elements are initialized with the term Initial instead of 0. Initial can contain other array definitions allowing thus for multi-dimensional arrays.
- g_array(List) : as above if List is a list of length Size except that the elements of the array are initialized according to the elements of List (which can contain other array definitions).
An array can be extended explicitly using a compound term with principal functor g_array_extend which accept the same 3 forms detailed above. In that case, the existing elements of the array are not initialized. If g_array_extend is used with an object which is not an array it is similar to g_array .
Finally, an array can be automatically expanded when needed. The programmer does not need to explicitly control the expansion of an automatic array. An array is expanded as soon as an index is outside the current size of this array. Such an array is defined using a compound term with principal functor g_array_auto :
- g_array_auto(Size) : if Size is an integer > 0 then defines an automatic array whose initial size is Size . All elements are initialized with the integer 0 . Elements created during implicit expansions will be initialized with 0 .
- g_array_auto(Size, Initial) : as above but the elements are initialized with the term Initial instead of 0. Initial can contain other array definitions allowing thus for multi-dimensional arrays. Elements created during implicit expansions will be initialized with Initial .
- g_array_auto(List) : as above if List is a list of length Size except that the elements of the array are initialized according to the elements of List (which can contain other array definitions). Elements created during implicit expansions will be initialized with 0 .
In any case, when an array is read, a term of the form g_array([Elem0,..., ElemSize-1]) is returned.
Some examples using global variables are presented later (section 8.21.7 ).
8.21.2 g_assign/2 , g_assignb/2 , g_link/2
Description
g_assign(GVarName, Value) assigns a copy of the term Value to GVarName . This assignment is not undone when backtracking occurs.
g_assignb/2 is similar to g_assign/2 but the assignment is undone at backtracking.
g_link(GVarName, Value) makes a link between GVarName to the term Value . This allows the user to give a name to any Prolog term (in particular non-ground terms). Such an assignment is always undone when backtracking occurs (since the term may no longer exist). If Value is an atom or an integer, g_link/2 and g_assignb/2 have the same behavior. Since g_link/2 only handles links to existing terms it does not require extra memory space and is not expensive in terms of execution time.
NB: argument selectors can only be used with g_assign/2 (i.e. when using an argument selector inside an assignment, this one must not be backtrackable).
See common errors detailed in the introduction (section 8.21.1 )
Portability
GNU Prolog predicates.
8.21.3 g_read/2
g_read(GVarName, Value) unifies Value with the term assigned to GVarName .
GNU Prolog predicate.
8.21.4 g_array_size/2
g_array_size(GVarName, Value) unifies Size with the dimension (an integer > 0) of the array assigned to GVarName . Fails if GVarName is not an array.
8.21.5 g_inc/3 , g_inc/2 , g_inco/2 , g_inc/1 , g_dec/3 , g_dec/2 , g_deco/2 , g_dec/1
g_inc(GVarName, Old, New) unifies Old with the integer assigned to GVarName , increments GVarName and then unifies New with the incremented value.
g_inc(GVarName, New) is equivalent to g_inc(GVarName, _, New) .
g_inco(GVarName, Old) is equivalent to g_inc(GVarName, Old, _) .
g_inc(GVarName) is equivalent to g_inc(GVarName, _, _) .
Predicates g_dec are similar but decrement the content of GVarName instead.
8.21.6 g_set_bit/2 , g_reset_bit/2 , g_test_set_bit/2 , g_test_reset_bit/2
g_set_bit(GVarName, Bit) sets to 1 the bit number specified by Bit of the integer assigned to GVarName to 1. Bit numbers range from 0 to the maximum number allowed for integers (this is architecture dependent). If Bit is greater than this limit, the modulo with this limit is taken.
g_reset_bit(GVarName, Bit) is similar to g_set_bit/2 but sets the specified bit to 0.
g_test_set_bit/2 succeeds if the specified bit is set to 1.
g_test_reset_bit/2 succeeds if the specified bit is set to 0.
8.21.7 Examples
Simulating g_inc/3 : this predicate behaves like: global variable:
The query: my_g_inc(c, X, _) will succeed unifying X with 0 , another call to my_g_inc(a, Y, _) will then unify Y with 1 , and so on.
Difference between g_assign/2 and g_assignb/2 : g_assign/2 does not undo its assignment when backtracking occurs whereas g_assignb/2 undoes it.
The query test(Old) will succeed unifying Old with 1 and on backtracking with 2 (i.e. the assignment of the value 2 has not been undone). The query testb(Old) will succeed unifying Old with 1 and on backtracking with 1 (i.e. the assignment of the value 2 has been undone).
Difference between g_assign/2 and g_link/2 : g_assign/2 (and g_assignb/2 ) creates a copy of the term whereas g_link/2 does not. g_link/2 can be used to avoid passing big data structures (e.g. dictionaries,…) as arguments to predicates.
The query test(B) will succeed unifying B with f(_) ( g_assign/2 assigns a copy of the value). The query test(B) will succeed unifying B with f(12) ( g_link/2 assigns a pointer to the term).
Simple array definition : here are some queries to show how arrays can be handled:
this is equivalent to:
2-D array definition :
Hybrid array :
Array extension :
Automatic array :
Introduction to Prolog
- First Online: 17 June 2023
Cite this chapter
- David S. Warren ORCID: orcid.org/0000-0001-7567-8156 13
Part of the book series: Lecture Notes in Computer Science ((LNAI,volume 13900))
756 Accesses
5 Citations
3 Altmetric
This first chapter of the Prolog50 book is brief introduction to the Prolog programming language. It is intended to provide background knowledge that will help in the understanding of many of the papers here. It covers basic Prolog definitions, their procedural interpretation, the idea of predicate modes, bottom-up evaluation of definitions, negation including stratified and nonstratified definitions, tabled evaluation, Prolog’s use of operators, program meta-interpretation, Definite Clause Grammars, and constraints. All topics are covered only briefly, and through the use of simple examples. For each topic there is much more to be said, some of which will be said in the papers in this volume.
This is a preview of subscription content, log in via an institution to check access.
Access this chapter
- Available as PDF
- Read on any device
- Instant download
- Own it forever
- Available as EPUB and PDF
- Compact, lightweight edition
- Dispatched in 3 to 5 business days
- Free shipping worldwide - see info
Tax calculation will be finalised at checkout
Purchases are for personal use only
Institutional subscriptions
Similar content being viewed by others
Logic Programming and Prolog
Semantics for Prolog with Cut – Revisited
The Prolog Debugger and Declarative Programming
We will see later that there may also be record structures.
Note this is a different meaning for a bound variable than in first-order logic.
See Chapter “Types, modes and so much more – the Prolog way,” in this volume.
See chapter “Ergo: A Quest for Declarativity in Logic Programming” for more on unstratified programs, and chapter “Prolog: Past, Present, and Future” for more on ASP and SMS.
Term expansion and other related facilities such as “attributed variables” have been used to add many other syntactic and semantic extensions to Prolog, such as functional programming and constraints. We discuss the latter in the following section.
See chapter “Introducing Prolog in Language-Informed Ways” for more uses of DCG’s.
See Fifty Years of Prolog and Beyond by Korner, Leuschel, Barbosa, Santos Costa, Dahl, Hermenegildo, Morales, Wielemaker, Diaz, and Abreu in TPLP 22(6).
Author information
Authors and affiliations.
Stony Brook University, Stony Brook, USA
David S. Warren
You can also search for this author in PubMed Google Scholar
Corresponding author
Correspondence to David S. Warren .
Editor information
Editors and affiliations.
Stony Brook University, Stony Brook, NY, USA
Simon Fraser University, Burnaby, BC, Canada
Veronica Dahl
TU Wien, Vienna, Austria
Thomas Eiter
Universidad Politecnica de Madrid/IMDEA Software Institute, Madrid, Spain
Manuel V. Hermenegildo
Imperial College London, London, UK
Robert Kowalski
IBM Research, Yorktown Heights, NY, USA
Francesca Rossi
Rights and permissions
Reprints and permissions
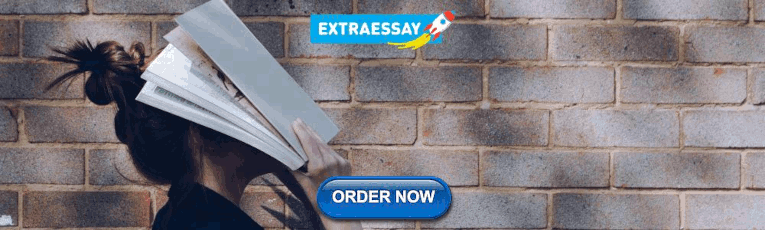
Copyright information
© 2023 The Author(s), under exclusive license to Springer Nature Switzerland AG
About this chapter
Warren, D.S. (2023). Introduction to Prolog. In: Warren, D.S., Dahl, V., Eiter, T., Hermenegildo, M.V., Kowalski, R., Rossi, F. (eds) Prolog: The Next 50 Years. Lecture Notes in Computer Science(), vol 13900. Springer, Cham. https://doi.org/10.1007/978-3-031-35254-6_1
Download citation
DOI : https://doi.org/10.1007/978-3-031-35254-6_1
Published : 17 June 2023
Publisher Name : Springer, Cham
Print ISBN : 978-3-031-35253-9
Online ISBN : 978-3-031-35254-6
eBook Packages : Computer Science Computer Science (R0)
Share this chapter
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
- Publish with us
Policies and ethics
- Find a journal
- Track your research
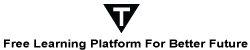
Computer Graphics
Computer Network
- Interview Q
Prolog Tutorial
Clauses & predicates, satisfying goals, operations & arithmetic, input & output, preventing backtracking, prolog examples.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

[ next ] [ prev ] [ prev-tail ] [ tail ] [ up ]
2.3 Arithmetic in Prolog
2.3.1 length/2, 2.3.2 exercises.
[ next ] [ prev ] [ prev-tail ] [ front ] [ up ]
Declarative Programming with Prolog – Part 2: Unification, Recursion, and Lists
July 5, 2018
Table of Contents
- Part 1 – Getting Started
- Part 2 – Unification, Recursion, and Lists
- Part 3 – Putting it All Together
Welcome back to this series on Declarative Programming with Prolog! If you haven’t already, make sure you check out the first post about getting started with Prolog, because in this post we’re going to dig deeper into some core concepts – specifically unification, recursion, and lists. Without further ado, let’s get into it.
Unification
We’ve already briefly mentioned unification and how we used it to set our variables in our rules and queries – but there’s more to it than that. At its core, unification means to make everything equal a valid result. This is different from assignment because we’re not usually telling Prolog exactly what our variables should equal – it’s figuring that out on its own.
Let’s use this example to understand more about unification:
The first two lines are nothing new – they’re just facts. We can even understand the next rule by now – especially now that you know unification is happening under the hood. When we query failed_houses with two variables, Prolog is unifying those variables to values that fit all of the subgoals – and as you can see, there are multiple combinations of values that satisfy them all:
Let’s take a look at that last rule now: little_pigs . What’s happening here is that we’re unifying the variables that are passed in to predetermined atoms – respectively straw , wood , and brick . This means that the rule will succeed only if we either pass all arguments as variables, or if we pass in arguments as atoms that already match those preset values:
However, the rule will fail if we try to pass in an atom that doesn’t match what we’re unifying the variables to:
Unification is a cool concept that’s core to how Prolog does its magic. The concepts below (as well as the last post in this series) will really help to illustrate unification more – keep following along!
Just like in many functional languages, Prolog doesn’t support formal looping constructs like for or while loops. This might seem like a limitation at first – but fear not; Prolog handles these needs with some snazzy recursion.
We’ll use this example to illustrate recursion in Prolog:
In this example, henry is a boss to rob , who is a boss to john , who is a boss to sam . We’re going to use recursion to illustrate a relationship between these 4 individuals beyond what we can query via the facts. To do this, we’re going to use two rules called higherup – each that accepts two arguments. It is completely valid to have facts and rules that have the same predicate and arity in Prolog – and only one of them ever needs to return true for the whole query to return true.
The first higherup rule is a base case that just checks if the two arguments have a direct boss fact that links them together.
The second higherup rule is where the real recursion happens; it accepts two arguments and tries to satisfy two subgoals: one which checks if X is a boss to someone , and another that checks if that same someone is a boss to Y. Confused yet? Let’s do an example
This query to the higherup rule will check the first rule it finds – which is our base case that checks if the two variables match to one of the boss facts. In this case, they do! The rule returns successfully, which means the whole query is true since only one successful rule or fact per predicate is needed. It never queries that second rule.
That was a pretty boring example – so let’s do another one:
Here is where the real recursion happens, and I’ll list out a chain of events to explain exactly what’s happening here:
- Prolog queries the higherup rule with the atoms henry and sam . The order matters.
- The first higherup rule fails because there’s no direct boss fact for henry and sam .
- The first subgoal unifies X to henry , and (we assume) Z to rob , since that’s currently the only atom with a common fact to henry in the order the arguments were passed.
- The second subgoal queries higherup again – this time passing in rob as the first argument and sam as the second.
- The first higherup rule fails again because there’s no direct boss fact for rob and sam .
- The first subgoal unifies X to rob , and (we assume) Z to john , since that’s currently the only atom with a common fact to rob in the order the arguments were passed.
- The second subgoal queries higherup again – this time passing in john as the first argument and sam as the second.
- The first higherup rule passes this time, because there IS a direct boss fact for john and sam !
- The whole query returns true.
Overall, it took three loops over our rules for our query to return successfully. You should be careful with recursion because as with most other languages, you can easily run out of application memory if you loop too many times. That is, unless you tail-call optimize your recursion, like we did in this example.
Tail-Call Optimization
Prolog (among many other languages) has the power of tail-call optimization during recursion which means that it’s possible to maintain constant memory usage throughout – no matter how many times you loop. You could loop 2 or 200 times – and memory usage would be the same. How is this possible, you might ask? This happens when your recursive action is the very last thing called in your rule (i.e. the tail call). In our example above, the very last subgoal of our very last rule with the higherup predicate is where our recursion happens – and every time it gets to this subgoal, Prolog only needs to remember one thing about the current stack frame: Is the query true up until this point? No logic will happen in the current stack frame after the recursive call – so it doesn’t need to store any data about the current stack frame because there’s no point in doing so. Each time Prolog comes across the recursive call, it knows that it can keep going deeper and all it has to remember is that the query is true up until that point. That means that when it finally does satisfy a base case or return false – it doesn’t pop all the way up the stack chain. It just flat out returns true or false right from where the query ended.
All of this goes out the window if you place another subgoal after the recursive call, or if you place another rule/fact with the same predicate and arity after the rule with the recursive action – because in that case, Prolog would need to store the state of the current stack frame so that it could resume logic at that point. For tail-call optimization to work, the recursive action has to be the last possible action in the current stack frame.
Lastly, we need to review how lists work. In Prolog, lists are containers of variable length, while tuples are containers of fixed length. Lists are indicated by brackets ( [] ) while tuples are indicated by parentheses ( () ).
While both certainly have their use-cases, we’ll focus solely on lists in this example because they can do some neat things that tuples can’t.
We can unify values inside of lists (as well as tuples):
You’ll notice that the variables are on the other side of the equals sign. This is probably weird to see – but remember that unification is different than assignment. Prolog uses unification to make sure that everything matches to a valid value – no matter where it is. We can even spread the variables on both sides of the equals sign:
Taking things a step further, we can deconstruct a list by splitting it into a head entry and another list that makes up everything but that first head entry:
This is something that tuples can’t do – and this becomes a really powerful concept behind what all you can do with lists. You can deconstruct a tail list even further:
In fact, if you wanted to get the n-th entry of a list, you would use list deconstruction until you got the entry you wanted. Here’s how we can get the third entry in a 5-length list:
The underscores are wildcard characters in Prolog, and they will accept any value and just toss it out; they’re practically just placeholders for values. Overall this looks ugly, right? I agree, and if you’re doing things like this, then that’s normally a code smell that you may not be using Prolog in the best way.
That’s all that we’re gonna cover with lists in Prolog; you can certainly get deeper than this – but there’s still really not much to them. We’ll showcase how you can use them in real-world examples in the next post.
Final thoughts
If I had to place emphasis on understanding one core concept in Prolog (other than basic facts, rules, and queries), it would be unification. No other language has this capability quite like Prolog does – and it’s really, really powerful (and not the easiest thing to understand on the first go through). With the power of unification, we’re able to build complex rules and queries that emphasize recursion, lists, math, and much more. I hope you enjoyed this post; we reviewed a lot – and we’re going to be using every bit of knowledge we gained here in the final post of this series where we’ll build a sudoku-solver with very little logic compared to how an imperative language might approach that problem.
Stay tuned!
Note: You can check out all the examples in this post in my Prolog Demo GitHub repo!
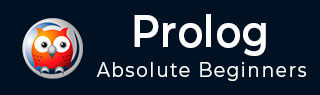
- Prolog Tutorial
- Prolog - Home
- Prolog - Introduction
- Prolog - Environment Setup
- Prolog - Hello World
- Prolog - Basics
- Prolog - Relations
- Prolog - Data Objects
Prolog - Operators
- Loop & Decision Making
- Conjunctions & Disjunctions
- Prolog - Lists
- Recursion and Structures
- Prolog - Backtracking
- Prolog - Different and Not
- Prolog - Inputs and Outputs
- Prolog - Built-In Predicates
- Tree Data Structure (Case Study)
- Prolog - Examples
- Prolog - Basic Programs
- Prolog - Examples of Cuts
- Towers of Hanoi Problem
- Prolog - Linked Lists
- Monkey and Banana Problem
- Prolog Useful Resources
- Prolog - Quick Guide
- Prolog - Useful Resources
- Prolog - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
In the following sections, we will see what are the different types of operators in Prolog. Types of the comparison operators and Arithmetic operators.
We will also see how these are different from any other high level language operators, how they are syntactically different, and how they are different in their work. Also we will see some practical demonstration to understand the usage of different operators.
Comparison Operators
Comparison operators are used to compare two equations or states. Following are different comparison operators −
You can see that the ‘=<’ operator, ‘=:=’ operator and ‘=\=’ operators are syntactically different from other languages. Let us see some practical demonstration to this.
Here we can see 1+2=:=2+1 is returning true, but 1+2=2+1 is returning false. This is because, in the first case it is checking whether the value of 1 + 2 is same as 2 + 1 or not, and the other one is checking whether two patterns ‘1+2’ and ‘2+1’ are same or not. As they are not same, it returns no (false). In the case of 1+A=B+2, A and B are two variables, and they are automatically assigned to some values that will match the pattern.
Arithmetic Operators in Prolog
Arithmetic operators are used to perform arithmetic operations. There are few different types of arithmetic operators as follows −
Let us see one practical code to understand the usage of these operators.
Note − The nl is used to create new line.
To Continue Learning Please Login
- Sources/building
- Docker images

- Command line
- Prolog syntax
- HTML generation
- Publications
- Rev 7 Extensions
- Getting started
- Development tools
- RDF namespaces
- GUI options
- Linux packages
- Report a bug
- Submit a patch
- Submit an add-on
- External links
- Contributing
- Code of Conduct
- Contributors
- SWI-Prolog items
- View changes
- cyclic_term/1
- acyclic_term/1
That naming is confusing
When you ask
you are actually asking whether the variable name X currently (at query time, a non-logical concept) designates and uninstantiated term, (aka. and unbound term, aka. an "empty storage cell").
The predicate would be less confusing if it had been named
However, an "empty storage cell" ( named by a variable name or unnamed and deep inside a term at some term tree leaf position) is traditionally called a "variable" or an "unbound variable". So be it.
"free" is also confusing
Note that the above text uses "free", which is even more confusing. "free" as an adjective applied to a variable means that said variable (in a first-order logic formula, a lambda expression etc.) is not bound by a quantifier (making the formula not well-formed). For example:
Y is free in the formula above.
On the other hand, the Mercury language uses the adjectives "free" and "bound"
Insts, modes, and mode definitions :
if the node is “free”, then the corresponding node in the term (if any) is a free variable that does not share with any other variable (we call such variables distinct); if the node is “bound”, then the corresponding node in the term (if any) is a function symbol.
Compare with Common Lisp's function
boundp checks whether the variable name passed as argument (and thus quoted: `'x`) is bound to something:
It behaves like nonvar/1 : `(boundp 'x)` evaluates to false if the symbol 'x designates an actual value. (It throws the argument is not actually a symbol).
Unlike in Prolog, you can make a bound symbol "unbound" again.
More on naming
Some notes on the ambiguity concerning the word "variable" can be found here
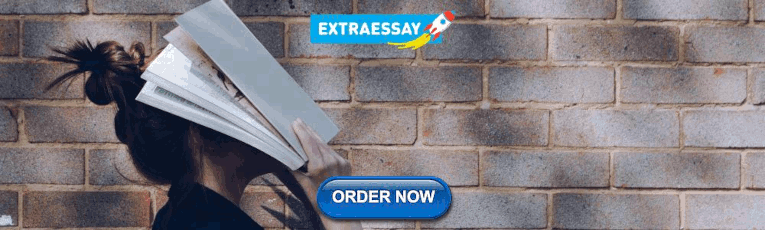
IMAGES
VIDEO
COMMENTS
Assign-once Variables. By saying that Prolog has assign-once variables, I mean that any particular variable in a Prolog procedure can only ever get one value assigned to it. A Prolog variable at any point in execution either has a value, which can thereafter never be changed, or it has not yet been given a value.
5. Variables in Prolog: All variables and arguments are local in scope to the predicate in which they are declared (aka first used). Excepting of course that variables may be passed as arguments (essentially "by reference") to another predicate. Prolog variables are only "variable" until bound (unified) with something else.
% Prolog makes magicNumber true by assigning one of the valid numbers to % the undefined variable Presto. By default it assigns the first one, 7. % By pressing ; in interactive mode you can reject that solution and % force it to assign the next one, 9.
In making a query you are asking Prolog whether it can prove that your query is true. If so, it answers "yes" and displays any variable bindings that it made in coming up with the answer. If it fails to prove the query true, it answers "No". Whenever you run the Prolog interpreter, it will prompt you with ?-. For example, suppose our database ...
z In PROLOG variables can besubstitutedwith certain values. This means that a variable can be assigned some value or beboundto it. z The assignment can be annulled as a result ofbacktrackingand then a new value can be assigned to the variable. z Once a value is assignedit cannot be overwritten!!!The variable must be free first. Example: WRONG!!!
GNU Prolog provides a simple and powerful way to assign and read global variables. A global variable is associated with each atom, its initial value is the integer 0. A global variable can store 3 kinds of objects: a copy of a term (the assignment can be made backtrackable or not). a link to a term (the assignment is always backtrackable).
Predicates that are true for some assignment of values to their variables are called satisfiable. Those that are true for all possible assignments of values to their variables are called valid. A prolog program is essentially an implementation of predicate logic. Prolog Syntax Prolog is based on facts, rules, queries, constants, and variables.
hasGrandparent is called, it is evaluated by first calling childOf with the indicated arguments, and then calling it again with different arguments (noting the calls do share the variable P). Variables in Prolog are assign-once variables, they either have a value or not, so once they get a value that value cannot change during procedure execution.
Data types. Prolog is dynamically typed.It has a single data type, the term, which has several subtypes: atoms, numbers, variables and compound terms.. An atom is a general-purpose name with no inherent meaning. It is composed of a sequence of characters that is parsed by the Prolog reader as a single unit. Atoms are usually bare words in Prolog code, written with no special syntax.
Of course, all variables of Prolog are essentially global (sometimes thread-local), or at least their content is - only the variables names exist in a specific namespace: the namespace of the clause. Any clause can access any term once one of its variable names has been bound to it. The "global variables" here so named are correctly described "variable names which exist in a specific global ...
Variables in Prolog. In the head or body of the clause, the variables are used. Variables are also used in goals, and those goals are entered at the system prompt. Variables in Goals. In goals, the variable can be interpreted as meaning 'find variable's value that makes the goal satisfied'. For example:
Prolog programs are built using predicates and clauses, with variables and constants forming the building blocks. Understanding the syntax and structure is crucial for writing effective Prolog ...
2.3. Arithmetic in Prolog. Prolog is not the programming language of choice for carrying out heavy-duty mathematics. It does, however, provide arithmetical capabilities. The pattern for evaluating arithmetic expressions is (where Expression is some arithmetical expression) X is Expression8. The variable X will be instantiated to the value of ...
You'll notice that the variables are on the other side of the equals sign. This is probably weird to see - but remember that unification is different than assignment. Prolog uses unification to make sure that everything matches to a valid value - no matter where it is. We can even spread the variables on both sides of the equals sign:
Prolog - Operators - In the following sections, we will see what are the different types of operators in Prolog. Types of the comparison operators and Arithmetic operators. ... In the case of 1+A=B+2, A and B are two variables, and they are automatically assigned to some values that will match the pattern. Arithmetic Operators in Prolog ...
Some corrections "A Prolog variable is a value that'is not yet bound'." That sound not correct (it would explain why var/1 is called var/1 and not unbound/1 though, but is in contradiction with all other usage of the word 'variable', in particular with the usage under "binding of a variable").. Better: A "variable" is a name (with clause scope) denoting a term or an empty cell.
If you want unnecessary parentheses anyway you can consider using atoms instead of terms as @lurker suggested. For example in SWI-Prolog, you can use term_to_atom/2 to convert an expression into an atom and atom_concat/2 to add '(' at the beginning and ')' at the end. But be aware that '(1*1)' does not unify with 1*1. And if you convert the ...
boundp checks whether the variable name passed as argument (and thus quoted: `'x`) is bound to something: (setq x 1) => 1. (boundp 'x) => true. (makunbound 'x) => X. (boundp 'x) => false. It behaves like nonvar/1: ` (boundp 'x)` evaluates to false if the symbol 'x designates an actual value. (It throws the argument is not actually a symbol ...
Prolog does not have assignment. True, you can give a variable a value. But you cannot change that value later; X = X + 1 is meaningless in mathematics and it's meaningless in Prolog too. In general, you will be working recursively, so you will simply create a new variable when something like this needs to occur.