Advisory boards aren’t only for executives. Join the LogRocket Content Advisory Board today →
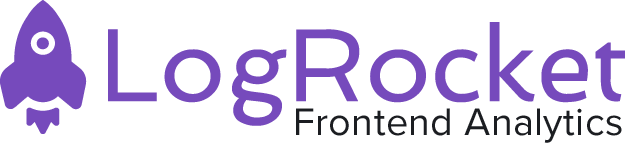
- Product Management
- Solve User-Reported Issues
- Find Issues Faster
- Optimize Conversion and Adoption
- Start Monitoring for Free
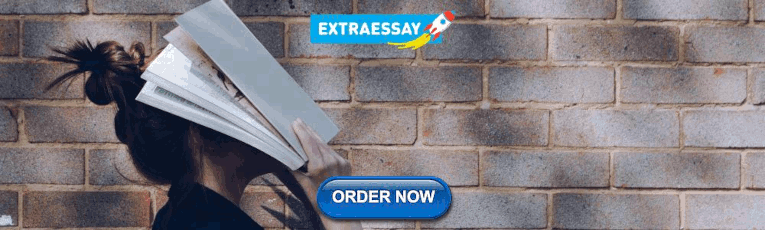
How to use pointers in Go
Go’s popularity has exploded in recent years. The 2020 HackerEarth Developer survey found that Go was the most sought-after programming language among experienced developers and students. The 2021 Stack Overflow Developer survey reported similar results, with Go being one of the top four languages that developers want to work with.
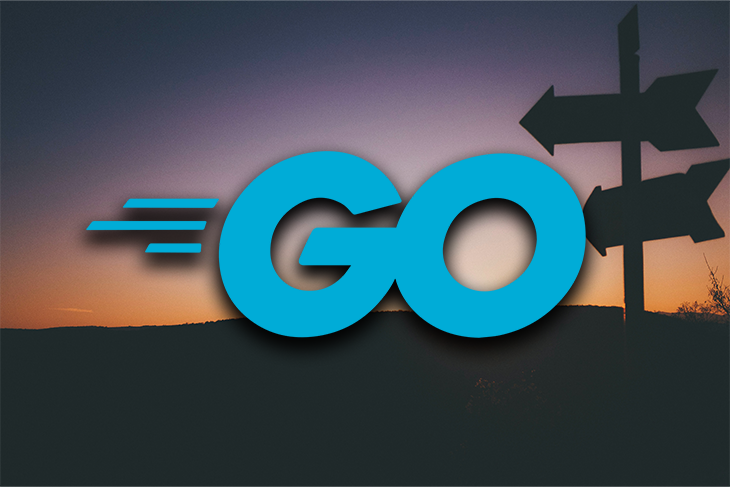
Given its popularity, it’s important for web developers to master Go, and perhaps one of the most critical components of Go is its pointers. This article will explain the different ways pointers can be created and the types of problems pointers fix.
What is Go?
Go is a statically typed, compiled language made by Google. There are many reasons why Go is such a popular choice for building robust, reliable, and efficient software. One of the biggest draws is Go’s simple and terse approach to writing software, which is apparent in the implementation of pointers in the language.
Passing arguments in Go
When writing software in any language, devs must consider what code could mutate in their codebase.
When you begin to compose functions and methods and pass around all different types of data structures in your code, you need to be careful of what should be passed by value and what should be passed by reference.
Passing an argument by value is like passing a printed copy of something. If the holder of the copy scribbles on it or destroys it, the original copy you have is unchanged.
Passing by reference is like sharing an original copy with someone. If they change something, you can see — and have to deal with — the changes they’ve made.
Let’s start with a really basic piece of code and see if you can spot why it might not be doing what we expect it to.
In the above example, I was trying to make the add10() function increment number 10 , but it doesn’t seem to be working. It just returns 0 . This is exactly the issue pointers solve.
Using pointers in Go
If we want to make the first code snippet work, we can make use of pointers.
In Go, every function argument is passed by value, meaning that the value is copied and passed, and by changing the argument value in the function body, nothing changes with the underlying variable.
The only exceptions to this rule are slices and maps. They can be passed by value and because they are reference types, any changes made to where they’re passed will change the underlying variable.
The way to pass arguments into functions that other languages consider “by reference” is by utilizing pointers.
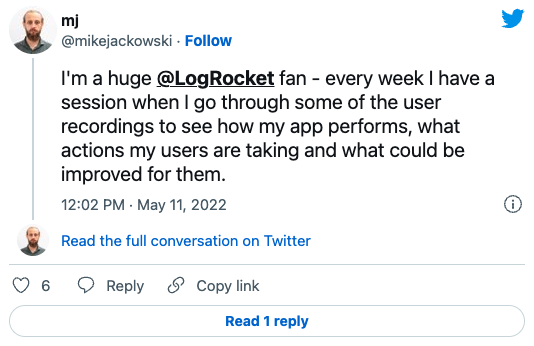
Over 200k developers use LogRocket to create better digital experiences

Let’s fix our first example and explain what’s happening.
Addressing pointer syntax
The only major difference between the first code snippet and the second was the usage of * and & . These two operators perform operations known as dereferencing/indirection ( * ) and referencing/memory address retrieval ( & ).
Referencing and memory address retrieval using &
If you follow the code snippet from the main function onward, the first operator we changed was to use an ampersand & in front of the number argument we passed into the add10 function.
This gets the memory address of where we stored the variable in the CPU. If you add a log to the first code snippet , you will see a memory address represented with hexadecimal. It will look something like this: 0xc000018030 (it will change each time you log).
This slightly cryptic string essentially points to an address on the CPU where your variable is stored. This is how Go shares the variable reference, so changes can be seen by all the other places that have access to the pointer or memory address.
Dereferencing memory using *
If the only thing we have now is a memory address, adding 10 to 0xc000018030 might not be exactly what we need. This is where dereferencing memory is useful.
We can, using the pointer, deference the memory address into the variable it points to, then do the math. We can see this in the above code snippet on line 14:
Here, we are dereferencing our memory address to 0 , then adding 10 to it.
Now the code example should work as initially expected. We share a single variable that changes are reflected against, and not by copying the value.
There are some extensions on the mental model we have created that will be helpful to understand pointers further.
Using nil pointers in Go
Everything in Go is given a 0 value when first initialized.
More great articles from LogRocket:
- Don't miss a moment with The Replay , a curated newsletter from LogRocket
- Learn how LogRocket's Galileo cuts through the noise to proactively resolve issues in your app
- Use React's useEffect to optimize your application's performance
- Switch between multiple versions of Node
- Discover how to use the React children prop with TypeScript
- Explore creating a custom mouse cursor with CSS
- Advisory boards aren’t just for executives. Join LogRocket’s Content Advisory Board. You’ll help inform the type of content we create and get access to exclusive meetups, social accreditation, and swag.
For example, when you create a string, it defaults to an empty string ( "" ) unless you assign something to it.
Here are all the zero values :
- 0 for all int types
- 0.0 for float32, float64, complex64, and complex128
- false for bool
- "" for string
- nil for interfaces, slices, channels, maps, pointers, and functions
This is the same for pointers. If you create a pointer but don’t point it to any memory address, it will be nil .
Using and dereferencing pointers
You can see here we were trying to re-use the ageOfSon variable in many places in our code, so we can just keep pointing things to other pointers.
But on line 15, we have to dereference one pointer, then dereference the next pointer it was pointing to.
This is utilizing the operator we already know, * , but it is also chaining the next pointer to be dereferenced, too.
This may seem confusing, but it will help that you have seen this ** syntax before when you look at other pointer implementations.
Creating a Go pointer with an alternate pointer syntax
The most common way to create pointers is to use the syntax that we discussed earlier. But there is also alternate syntax you can use to create pointers using the new() function.
Let’s look at an example code snippet .
The syntax is only slightly different, but all of the principles we have already discussed are the same.
Common Go pointer misconceptions
To review everything we’ve learned, there are some often-repeated misconceptions when using pointers that are useful to discuss.
One commonly repeated phrase whenever pointers are discussed is that they’re more performant, which, intuitively, makes sense.
If you passed a large struct, for example, into multiple different function calls, you can see how copying that struct multiple times into the different functions might slow down the performance of your program.
But passing pointers in Go is often slower than passing copied values.
This is because when pointers are passed into functions, Go needs to perform an escape analysis to work out whether the value needs to be stored on the stack or in the heap.
Passing by value allows all the variables to be stored on the stack, which means garbage collection can be skipped for that variable.
Check out this example program here :
When allocating one billion pointers, the garbage collector can take over half a second. This is less than a nanosecond per pointer. But it can add up, especially when pointers are used this heavily in a huge codebase with intense memory requirements.
If you use the same code above without using pointers, the garbage collector can run more than 1,000 times faster .
Please test the performance of your use cases, as there are no hard and fast rules. Just remember the mantra, “Pointers are always faster,” is not true in every scenario.
I hope this has been a useful summary. In it, we covered what Go pointers are, different ways they can be created, what problems they solve, as well as some issues to be aware of in their use cases.
When I first learned about pointers, I read a multitude of well-written, large codebases on GitHub (like Docker for example) to try and understand when and when not to use pointers, and I encourage you to do the same.
It was very helpful to consolidate my knowledge and understand in a hands-on way the different approaches teams take to use pointers to their fullest potential.
There are many questions to consider, such as:
- What do our performance tests indicate?
- What is the overall convention in the wider codebase?
- Does this make sense for this particular use case?
- Is it simple to read and understand what is happening here?
Deciding when and how to use pointers is on a case-by-case basis, and I hope you now have a thorough understanding of when to best utilize pointers in your projects.
Get set up with LogRocket's modern error tracking in minutes:
- Visit https://logrocket.com/signup/ to get an app ID
Install LogRocket via npm or script tag. LogRocket.init() must be called client-side, not server-side
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Facebook (Opens in new window)
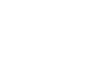
Stop guessing about your digital experience with LogRocket
Recent posts:.
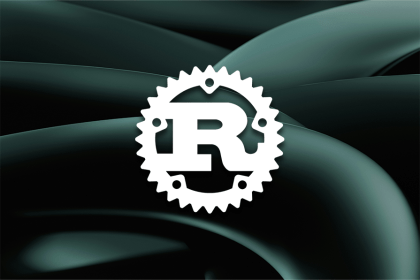
Using Pavex for Rust web development
The Pavex Rust web framework is an exciting project that provides high performance, great usability, and speed.
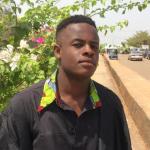
Using the ResizeObserver API in React for responsive designs
With ResizeObserver, you can build aesthetic React apps with responsive components that look and behave as you intend on any device.
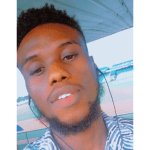
Creating JavaScript tables using Tabulator
Explore React Tabulator to create interactive JavaScript tables, easily integrating pagination, search functionality, and bulk data submission.
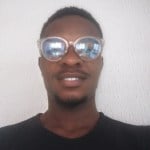
How to create heatmaps in JavaScript: The Heat.js library
This tutorial will explore the application of heatmaps in JavaScript projects, focusing on how to use the Heat.js library to generate them.

Leave a Reply Cancel reply
Pointers in Golang – 10+ Things You MUST Know
Pointers are one of the most important building blocks of writing good code. In this post, we are going to explore pointers, what they are, and how can they be used in Go to write excellent code.
1. What is a pointer?
A pointer is a variable that stores the address it points to. A pointer of a specific type can only point to that type.
2. Golang Pointer syntax
The syntax for the pointers is really simple. Here is the syntax of the declaration of pointers in Go.
The zero-value of the pointer is nil .
3. Pointers Initialization in Go
The pointers of a type are initialized using the address-of(&) operator on a subject of that specific type. Here is the way to do it.
4. Go Pointers dereferencing
Dereferencing a pointer means getting the value inside the address the pointer holds. If we have a memory address, we can dereference the pointer to that memory address to get the value inside it. Here is the same example showing the dereference operation using the star(*) operator.
5. Pointer to pointer in Golang
A pointer variable can store even a pointers address since a pointer is also a variable just like others. So, we can point to a pointer and create levels of indirection. These levels of indirection can sometimes create unnecessary confusion so be wary when using it.
6. Pointer to interfaces
A pointer can point to anything even an interface. When an empty interface is used it returns nil as a value.
Here is an example using an interface with pointers.
Here “ a” is a struct of the type Bird which is then used for an interface type as you can see. This is polymorphism in action. Go allows polymorphism using interfaces . So, you can see pointers to a struct or an interface is an essential tool in Go.
7. Pointers as function arguments
Pointers can be used in function arguments just like value. It has some advantages over using values directly. It is a very efficient way to pass large objects to function. So, it is a great optimization to have it.
Using large objects can slow down execution time, here is an example of passing a pointer to a struct. This is an efficient way to handle large objects.
Be careful when dereferencing a struct. If you use it like *structname.field1 then it will throw an error. The correct way to do it is (*structname).field1 .
Using pointers inside functions makes the value mutable unless its const . So, whenever we want to change a value we should use a pointer to that as function argument and then do necessary modifications.
8. The “new” function in Go
The new function in Go returns a pointer to a type.
9. Returning a pointer from a function
Pointers of any type can be returned from a function just like any other value. It is really simple. Instead of returning the value directly we simply return the address of that value.
10. Pointers to a function
Pointers to a function work implicitly in Go. That means we don’t need to declare it as a pointer.
11. Things to keep in mind while using Pointers in Go
Go doesn’t allow pointer arithmetic. So, we cannot do anything like unary increment or decrement as we can do in C/C++.
We may want to use a pointer to an array but there is a better option for it. And that’s slices . Slices are much more versatile than a pointer to an array. The code is concise and makes our lives a lot easier. So, use slices whenever possible.
Exploring Golang Pointers: A Deep Dive
Pointers are a powerful feature in the Go programming language that allow you to work directly with memory addresses. Understanding pointers is essential for writing efficient and idiomatic Go code. In this blog post, we'll take a comprehensive look at pointers in Go, covering their syntax, usage, and common pitfalls.
Introduction to Pointers
In Go, a pointer is a variable that stores the memory address of another variable. This allows you to indirectly access and modify the value of the variable through its pointer. Pointers are commonly used in Go for passing large data structures efficiently and for implementing data structures like linked lists and trees.
The syntax for declaring a pointer variable in Go is as follows:
This declares a pointer variable ptr that can hold the memory address of an integer variable.
Using Pointers in Go
Initializing pointers.
Pointers can be initialized using the address-of operator ( & ) followed by the variable whose address you want to store.
Dereferencing Pointers
Dereferencing a pointer means accessing the value stored at the memory address held by the pointer. This is done using the dereference operator ( * ).
Passing Pointers to Functions
Pointers are commonly used to pass variables by reference to functions, allowing functions to modify the original value of the variable.
Returning Pointers from Functions
Functions can also return pointers, allowing them to return the address of a variable allocated within the function.
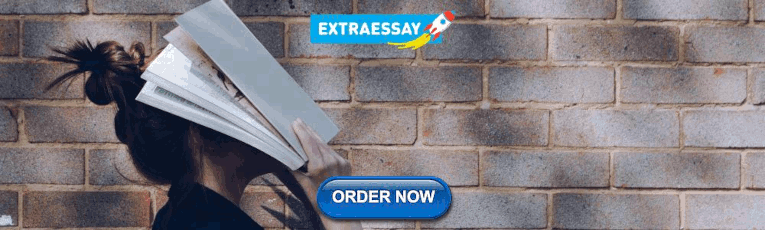
Pitfalls and Best Practices
Null pointers.
Unlike some other languages, Go does not have null pointers. However, uninitialized pointers have a zero value, which is nil .
Lifetime of Pointers
Avoid returning pointers to local variables from functions, as the memory allocated to local variables is deallocated once the function returns.
Pointers are a powerful feature in Go that allow for more efficient memory management and can be used to create complex data structures. Understanding how pointers work and how to use them correctly is essential for writing idiomatic Go code. By following the guidelines outlined in this blog post, you can leverage pointers effectively in your Go programs.
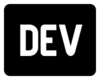
DEV Community

Posted on May 17, 2023
Golang Pointers and Functions: A Guide with Examples
In this article, we'll explore how Golang pointers work in functions, and we'll provide examples of using them with and without memory references.
What are Pointers in Golang?
A pointer is a variable that contains the memory address of another variable. In Golang, a pointer is represented by an asterisk (*) followed by the data type of the variable it points to. For example, the following code declares a pointer to an integer variable:
This declares a pointer named "ptr" that points to an integer variable. To assign the address of a variable to a pointer, use the "&" operator. For example:
This assigns the address of the "num" variable to the "ptr" pointer. Now, the "ptr" pointer points to the "num" variable in memory.
Using Pointers in Functions
When a pointer is passed to a function, the function can modify the value of the variable it points to directly in memory. This is much more efficient than passing a copy of the variable to the function, which requires creating a new variable in memory.
Let's take a look at an example:
This function takes a pointer to a string variable as its argument. Inside the function, we use the "*" operator to dereference the pointer and access the value of the variable it points to. Then, we can modify the value of the variable directly in memory by using the "=" assignment operator.
With Memory References
Now, let's take a look at an example of using a pointer with a memory reference:
In this example, we declare a string variable named "chain" and assign it the value "Hello world". We then print the memory address of the "chain" variable using the "&" operator.
Next, we call the "updateValueWithRef" function and pass it a reference to the "chain" variable using the "&" operator. Inside the function, we modify the value of the variable directly in memory using the "*" operator.
After the function call, we print the value of the "chain" variable again, and we can see that it has been modified by the function.
Without Memory References
To understand the difference between using a pointer with and without memory references, let's take a look at an example of using a pointer without a memory reference:
In this function, we take a string variable as its argument. Inside the function, we modify the value of the variable using the "=" assignment operator.
Now, let's call this function instead of the "updateValueWithRef" function in our previous example:
In this example, we call the "updateValue" function and pass it a copy of the "chain" variable. Inside the function, we modify the value of the copy using the "=" assignment operator.
After the function call, we print the value of the "chain" variable again, and we can see that it has not been modified by the function.
Full code example:
In conclusion, pointers are a powerful feature of Golang that enable developers to write more efficient code by manipulating data directly in memory. When using pointers in functions, it's important to understand the difference between using them with and without memory references, as this can affect how the function modifies the value of the variable it points to.
By understanding how pointers and functions work together in Golang, developers can write more efficient and scalable code that takes advantage of the language's unique features.
Top comments (1)
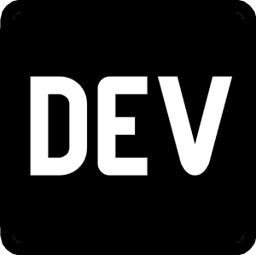
Templates let you quickly answer FAQs or store snippets for re-use.

- Location Indonesia
- Work BackEnd Engineer At BlueBird Group
- Joined Dec 3, 2020
thanks for sharing
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
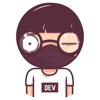
How to get CMS in any Next app with one line
Anmol Baranwal - May 8

The fastest way to concatenate strings in Golang
Shubham Chadokar - Apr 30
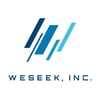
Building a Docker Development Environment (Part 1)
Weseek-Inc - Apr 30
Building a Docker Development Environment (Part 2)
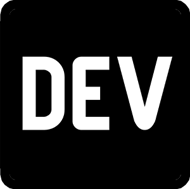
We're a place where coders share, stay up-to-date and grow their careers.
Popular Tutorials
Popular examples, learn python interactively, go introduction.
- Golang Getting Started
- Go Variables
- Go Data Types
- Go Print Statement
- Go Take Input
- Go Comments
- Go Operators
- Go Type Casting
Go Flow Control
- Go Boolean Expression
- Go if...else
- Go for Loop
- Go while Loop
- Go break and continue
Go Data Structures
- Go Functions
- Go Variable Scope
- Go Recursion
- Go Anonymous Function
- Go Packages
Go Pointers & Interface
Go Pointers
- Go Pointers and Functions
- Go Pointers to Struct
Go Interface
- Go Empty Interface
- Go Type Assertions
Go Additional Topics
- Go defer, panic, and recover
Go Tutorials
Go pointers to structs.
The struct types store the variables of different data types and we use the struct variable to access the members of the struct.
In Go, we can also create a pointer variable of struct type.
Before you learn about pointers to struct, make sure to know about:
- Go Pointer to Struct
Suppose we have a struct like this
Now, let's create a struct variable of Person type.
Similar to this, we can also create a pointer variable of struct type.
We can now assign the address of the struct variable to this pointer variable. Let's see an example.
In the above example, we have created a struct variable person1 that initialized the struct members; name to John and age to 25 .
We have also created a pointer variable of the struct type that stores the address of person1 .
Since the ptr now stores the address of person1 , we get &{John 25} as the output while printing ptr .
Note : We can also create struct-type pointers and assign variable addresses in the same line. For example,
Access struct using pointer in Golang
We can also access the individual member of a struct using the pointer. For example,
In the above example, we have used the struct type pointer to access struct members:
- ptr.name - gives the value of the name member
- ptr.age - gives the value of the age member
Here, we have used the dot operator to access the struct members using the pointer.
Note: We can also use the dereference operator, * to access the members of struct. For example,
Change the Struct member in Go
Similarly, we can also use the pointer variable and the dot operator to change the value of a struct member. For example,
In the above example, notice the line
Here, we have changed the value of the struct member temperature to 25 using the pointer variable ptr .
Table of Contents
- Introduction
- Access struct using pointer
- Change the Struct member
Sorry about that.
Related Tutorials
Programming
Go by Example : Pointers
Next example: Strings and Runes .
by Mark McGranaghan and Eli Bendersky | source | license
Welcome to tutorial no. 15 in Golang tutorial series .
In this tutorial, we will learn how pointers work in Go and we will also understand how Go pointers differ from pointers in other languages such as C and C++.
This tutorial has the following sections.
What is a pointer?
Declaring pointers, zero value of a pointer, creating pointers using the new function, dereferencing a pointer, passing pointer to a function, returning pointer from a function, do not pass a pointer to an array as an argument to a function. use slice instead., go does not support pointer arithmetic.
A pointer is a variable that stores the memory address of another variable.
In the above illustration, variable b has value 156 and is stored at memory address 0x1040a124 . The variable a holds the address of b . Now a is said to point to b .
* T is the type of the pointer variable which points to a value of type T .
Let’s write a program that declares a pointer.
Run in playground
The & operator is used to get the address of a variable. In line no. 9 of the above program we are assigning the address of b to a whose type is *int . Now a is said to point to b. When we print the value in a , the address of b will be printed. This program outputs
You might get a different address for b since the location of b can be anywhere in memory.
The zero value of a pointer is nil .
b is initially nil in the above program and then later it is assigned to the address of a. This program outputs
Go also provides a handy function new to create pointers. The new function takes a type as an argument and returns a pointer to a newly allocated zero value of the type passed as argument.
The following example will make things more clear.
In the above program, in line no. 8 we use the new function to create a pointer of type int . This function will return a pointer to a newly allocated zero value of the type int . The zero value of type int is 0 . Hence size will be of type *int and will point to 0 i.e *size will be 0 .
The above program will print
Dereferencing a pointer means accessing the value of the variable to which the pointer points. *a is the syntax to deference a.
Let’s see how this works in a program.
In line no 10 of the above program, we deference a and print the value of it. As expected it prints the value of b. The output of the program is
Let’s write one more program where we change the value in b using the pointer.
In line no. 12 of the above program, we increment the value pointed by a by 1 which changes the value of b since a points to b . Hence the value of b becomes 256. The output of the program is
In the above program, in line no. 14 we are passing the pointer variable b which holds the address of a to the function change . Inside change function, the value of a is changed using dereference in line no 8. This program outputs,
It is perfectly legal for a function to return a pointer of a local variable. The Go compiler is intelligent enough and it will allocate this variable on the heap.
In line no. 9 of the program above, we return the address of the local variable i from the function hello . The behaviour of this code is undefined in programming languages such as C and C++ as the variable i goes out of scope once the function hello returns. But in the case of Go, the compiler does an escape analysis and allocates i on the heap as the address escapes the local scope. Hence this program will work and it will print,
Let’s assume that we want to make some modifications to an array inside the function and the changes made to that array inside the function should be visible to the caller. One way of doing this is to pass a pointer to an array as an argument to the function.
In line no. 13 of the above program, we are passing the address of the array a to the modify function. In line no.8 in the modify function we are dereferencing arr and assigning 90 to the first element of the array. This program outputs [90 90 91]
a[x] is shorthand for (*a)[x]. So (*arr)[0] in the above program can be replaced by arr[0] . Let’s rewrite the above program using this shorthand syntax.
This program also outputs [90 90 91]
Although this way of passing a pointer to an array as an argument to a function and making modification to it works, it is not the idiomatic way of achieving this in Go. We have slices for this.
Let’s rewrite the same program using slices .
In line no.13 of the program above, we pass a slice to the modify function. The first element of the slice is changed to 90 inside the modify function. This program also outputs [90 90 91] . So forget about passing pointers to arrays around and use slices instead :) . This code is much cleaner and is idiomatic Go :).
Go does not support pointer arithmetic which is present in other languages like C and C++.
The above program will throw compilation error * main.go:6: invalid operation: p++ (non-numeric type [3]int)
I have created a single program in github which covers everything we have discussed.
That’s it for pointers in Go. I hope you liked this tutorial. Please leave your feedback and comments. Please consider sharing this tutorial on twitter and LinkedIn . Have a good day.
If you would like to advertise on this website, hire me, or if you have any other software development needs please email me at naveen[at]golangbot[dot]com .
Next tutorial - Structs
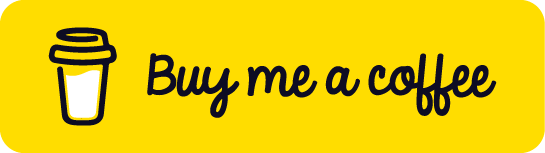
- Data Types in Go
- Go Keywords
- Go Control Flow
- Go Functions
- GoLang Structures
- GoLang Arrays
- GoLang Strings
- GoLang Pointers
- GoLang Interface
- GoLang Concurrency
- Go Programming Language (Introduction)
- How to Install Go on Windows?
- How to Install Golang on MacOS?
- Hello World in Golang
Fundamentals
- Identifiers in Go Language
- Go Variables
- Constants- Go Language
- Go Operators
Control Statements
- Go Decision Making (if, if-else, Nested-if, if-else-if)
- Loops in Go Language
- Switch Statement in Go
Functions & Methods
- Functions in Go Language
- Variadic Functions in Go
- Anonymous function in Go Language
- main and init function in Golang
- What is Blank Identifier(underscore) in Golang?
- Defer Keyword in Golang
- Methods in Golang
- Structures in Golang
- Nested Structure in Golang
- Anonymous Structure and Field in Golang
- Arrays in Go
- How to Copy an Array into Another Array in Golang?
- How to pass an Array to a Function in Golang?
- Slices in Golang
- Slice Composite Literal in Go
- How to sort a slice of ints in Golang?
- How to trim a slice of bytes in Golang?
- How to split a slice of bytes in Golang?
- Strings in Golang
- How to Trim a String in Golang?
- How to Split a String in Golang?
- Different ways to compare Strings in Golang
Pointers in Golang
- Passing Pointers to a Function in Go
- Pointer to a Struct in Golang
- Go Pointer to Pointer (Double Pointer)
- Comparing Pointers in Golang
Concurrency
- Goroutines - Concurrency in Golang
- Select Statement in Go Language
- Multiple Goroutines
- Channel in Golang
- Unidirectional Channel in Golang
Pointers in Go programming language or Golang is a variable that is used to store the memory address of another variable. Pointers in Golang is also termed as the special variables. The variables are used to store some data at a particular memory address in the system. The memory address is always found in hexadecimal format(starting with 0x like 0xFFAAF etc.).
What is the need for the pointers?
To understand this need, first, we have to understand the concept of variables. Variables are the names given to a memory location where the actual data is stored. To access the stored data we need the address of that particular memory location. To remember all the memory addresses(Hexadecimal Format) manually is an overhead that’s why we use variables to store data and variables can be accessed just by using their name. Golang also allows saving a hexadecimal number into a variable using the literal expression i.e. number starting from 0x is a hexadecimal number.
Example: In the below program, we are storing the hexadecimal number into a variable. But you can see that the type of values is int and saved as the decimal or you can say the decimal value of type int is storing. But the main point to explain this example is that we are storing a hexadecimal value(consider it a memory address) but it is not a pointer as it is not pointing to any other memory location of another variable. It is just a user-defined variable. So this arises the need for pointers.
Output:
A pointer is a special kind of variable that is not only used to store the memory addresses of other variables but also points where the memory is located and provides ways to find out the value stored at that memory location. It is generally termed as a Special kind of Variable because it is almost declared as a variable but with * (dereferencing operator).
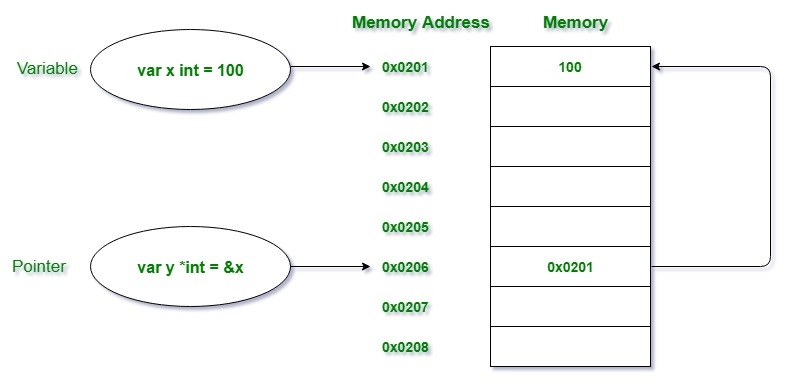
Declaration and Initialization of Pointers
Before we start there are two important operators which we will use in pointers i.e.
- * Operator also termed as the dereferencing operator used to declare pointer variable and access the value stored in the address.
- & operator termed as address operator used to returns the address of a variable or to access the address of a variable to a pointer.
Declaring a pointer :
Example: Below is a pointer of type string which can store only the memory addresses of string variables.
Initialization of Pointer: To do this you need to initialize a pointer with the memory address of another variable using the address operator as shown in the below example:
Output:
Important Points
1. The default value or zero-value of a pointer is always nil . Or you can say that an uninitialized pointer will always have a nil value.
2. Declaration and initialization of the pointers can be done into a single line.
Example:
3. If you are specifying the data type along with the pointer declaration then the pointer will be able to handle the memory address of that specified data type variable. For example, if you taking a pointer of string type then the address of the variable that you will give to a pointer will be only of string data type variable, not any other type.
4. To overcome the above mention problem you can use the Type Inference concept of the var keyword . There is no need to specify the data type during the declaration. The type of a pointer variable can also be determined by the compiler like a normal variable. Here we will not use the * operator. It will internally determine by the compiler as we are initializing the variable with the address of another variable.
5. You can also use the shorthand (:=) syntax to declare and initialize the pointer variables. The compiler will internally determine the variable is a pointer variable if we are passing the address of the variable using &(address) operator to it.
Dereferencing the Pointer
As we know that * operator is also termed as the dereferencing operator. It is not only used to declare the pointer variable but also used to access the value stored in the variable which the pointer points to which is generally termed as indirecting or dereferencing . * operator is also termed as the value at the address of . Let’s take an example to get a better understandability of this concept:
Output:
You can also change the value of the pointer or at the memory location instead of assigning a new value to the variable.
Please Login to comment...
Similar reads.
- Golang-Pointers
- Go Language
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Is assigning a pointer atomic in go #38667
SmallSmartMouse commented Apr 26, 2020
randall77 commented Apr 26, 2020
Sorry, something went wrong.
SmallSmartMouse commented Apr 29, 2020
lsytj0413 commented Apr 29, 2020
ianlancetaylor commented Apr 30, 2020
No branches or pull requests
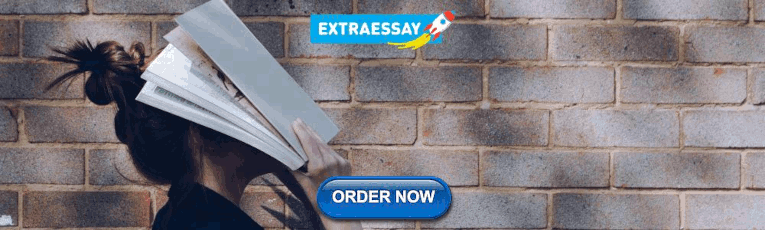
IMAGES
VIDEO
COMMENTS
The pointer doesn't actually contain an int32, just the address of one. Let's take a look at a pointer to a string. The following code declares both a value of a string, and a pointer to a string: ... (or GoLang) is a modern programming language originally developed by Google that uses high-level syntax similar to scripting languages. It is ...
I'm trying to assign a value to a struct member that is a pointer, but it gives "panic: runtime error: invalid memory address or nil pointer dereference" at runtime... package main import ( "fmt" "strconv" ) // Test type stctTest struct { blTest *bool } func main() { var strctTest stctTest *strctTest.blTest = false fmt.Println("Test is ...
Creating a Go pointer with an alternate pointer syntax. The most common way to create pointers is to use the syntax that we discussed earlier. But there is also alternate syntax you can use to create pointers using the new() function. Let's look at an example code snippet. package main.
Go Pointer Variables. In Go, we use the pointer variables to store the memory address. For example, var num int = 5 // create the pointer variable var ptr *int = &num. Here, we have created the pointer variable named ptr that stores the memory address of the num variable. *int represents that the pointer variable is of int type (stores the ...
Pointers are a powerful feature of Go that allow you to manipulate memory addresses and access data directly in memory. They are commonly used to create references to variables, pass arguments to ...
Here is the syntax of the declaration of pointers in Go. 1. 2. var ptr *type. var ptrint *int // pointer to an int. The zero-value of the pointer is nil. 3. Pointers Initialization in Go. The pointers of a type are initialized using the address-of (&) operator on a subject of that specific type.
Introduction to Pointers. In Go, a pointer is a variable that stores the memory address of another variable. This allows you to indirectly access and modify the value of the variable through its pointer. Pointers are commonly used in Go for passing large data structures efficiently and for implementing data structures like linked lists and trees.
To assign the address of a variable to a pointer, use the "&" operator. For example: var num int = 42. ptr = &num. This assigns the address of the "num" variable to the "ptr" pointer. Now, the "ptr" pointer points to the "num" variable in memory. Using Pointers in Functions. When a pointer is passed to a function, the function can modify the ...
Access struct using pointer in Golang. We can also access the individual member of a struct using the pointer. For example, // Program to access the field of a struct using pointer package main import "fmt" func main() { // declare a struct Person type Person struct { name string age int } person := Person{"John", 25} // create a struct type pointer that // stores the address of person var ptr ...
Go supports pointers, allowing you to pass references to values and records within your program.. package main: import "fmt": We'll show how pointers work in contrast to values with 2 functions: zeroval and zeroptr.zeroval has an int parameter, so arguments will be passed to it by value.zeroval will get a copy of ival distinct from the one in the calling function.
go. Run in playground. In the above program, in line no. 8 we use the new function to create a pointer of type int. This function will return a pointer to a newly allocated zero value of the type int. The zero value of type int is 0. Hence size will be of type *int and will point to 0 i.e *size will be 0.
Pointers. Go has pointers. A pointer holds the memory address of a value. The type *T is a pointer to a T value. Its zero value is nil.. var p *int. The & operator generates a pointer to its operand.. i := 42 p = &i. The * operator denotes the pointer's underlying value.. fmt.Println(*p) // read i through the pointer p *p = 21 // set i through the pointer p
Pointers in Go programming language or Golang is a variable that is used to store the memory address of another variable. Pointers in Golang is also termed as the special variables. The variables are used to store some data at a particular memory address in the system. The memory address is always found in hexadecimal format (starting with 0x ...
The answer to the former, as of right now is yes, assigning (loading/storing) a pointer is atomic in Golang, this lies in the updated Go memory model Otherwise, a read r of a memory location x that is not larger than a machine word must observe some write w such that r does not happen before w and there is no write w' such that w happens before ...
Remember: every variable has an address, and the value of a pointer is the address. In the C language, the most complained about pointers is the operation of pointers and memory release, which often lead to some buffer overflow problems. The concept of pointers is also retained in Golang, but pointer arithmetic cannot be performed.
Instead of trying to do math with the pointer itself, we need the value that the pointer points to. Because a pointer is a reference to some variable, the fancy name for this is dereferencingthe pointer. To get the value pointed to by input, we write *input("star-input"): *input *= 2. Nil pointers and panics.
Introduction. In the vast universe of Go (Golang), pointers play a pivotal role in developing efficient and precise code. This comprehensive guide aims to provide an in-depth understanding of pointers in Go, from the basics to advanced applications, with the goal of equipping developers with the skills necessary to write robust and optimized code.
There are two reasons to use a pointer receiver. The first is so that the method can modify the value that its receiver points to. The second is to avoid copying the value on each method call. This can be more efficient if the receiver is a large struct, for example. In this example, both Scale and Abs are methods with receiver type *Vertex ...
71. In the first example: s = &student{"unknown", 0} You are assigning an entirely new "pointer value" to s, and the new *s points at a new student value. The variable s is scoped only to the method body, so there are no side effects after this returns. In the second example. *s = student{"unknown", 0}
More generally, pointer assignment is atomic, but you need more than that to make an example like yours work. ... golang locked and limited conversation to collaborators Apr 30, 2021. gopherbot added the FrozenDueToAge label Apr 30, 2021.
In Obj-C, atomic properties will ensure operations are performed one after another, to prevent threads from accessing a memory address at the same time. Since Go is multithreaded, it supports atomic operations as well. Go 1.19 introduces new atomic types. My favorite addition is atomic.Pointer , it provides a sleek alternative to atomic.Value .